I absolutely love tree problems!
It had been a while since I had seen a fresh tree problem on Leetcode. This is a Medium one, but fairly straightforward - a direct implementation of a post-order depth-first-search traversal. Code is down below, cheers, ACC.
Count Nodes Equal to Sum of Descendants - LeetCode
1973. Count Nodes Equal to Sum of Descendants
Medium
Given the root
of a binary tree, return the number of nodes where the value of the node is equal to the sum of the values of its descendants.
A descendant of a node x
is any node that is on the path from node x
to some leaf node. The sum is considered to be 0
if the node has no descendants.
Example 1:
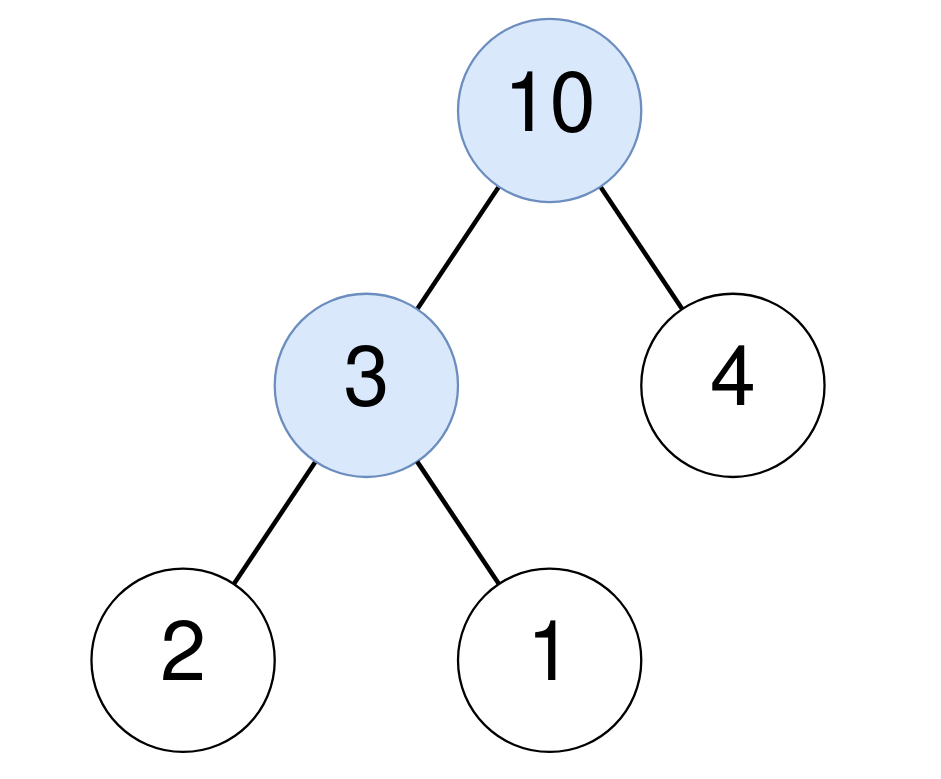
Input: root = [10,3,4,2,1] Output: 2 Explanation: For the node with value 10: The sum of its descendants is 3+4+2+1 = 10. For the node with value 3: The sum of its descendants is 2+1 = 3.
Example 2:
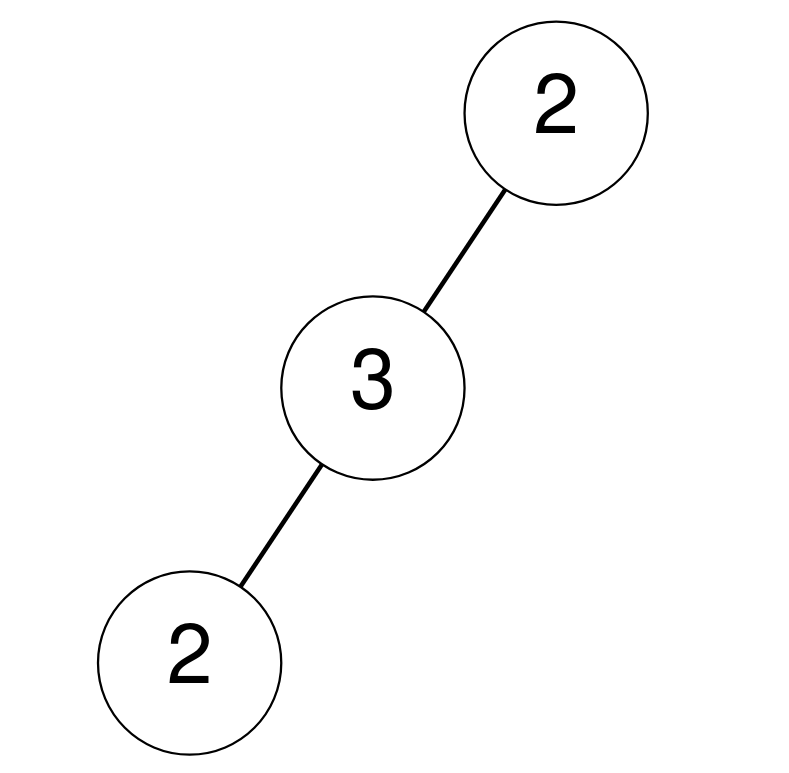
Input: root = [2,3,null,2,null] Output: 0 Explanation: No node has a value that is equal to the sum of its descendants.
Example 3:
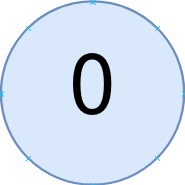
Input: root = [0] Output: 1 For the node with value 0: The sum of its descendants is 0 since it has no descendants.
Constraints:
- The number of nodes in the tree is in the range
[1, 105]
. 0 <= Node.val <= 105
public int EqualToDescendants(TreeNode root) { int sumDescendants = 0; int retVal = 0; EqualToDescendants(root, ref sumDescendants, ref retVal); return retVal; } private void EqualToDescendants(TreeNode currentNode, ref int sumDescendants, ref int retVal) { if (currentNode == null) { sumDescendants = 0; return; } int sumDescendantsLeft = 0; EqualToDescendants(currentNode.left, ref sumDescendantsLeft, ref retVal); int sumDescendantsRight = 0; EqualToDescendants(currentNode.right, ref sumDescendantsRight, ref retVal); if (currentNode.val == sumDescendantsLeft + sumDescendantsRight) { retVal++; } sumDescendants = currentNode.val + sumDescendantsLeft + sumDescendantsRight; }
Comments
Post a Comment